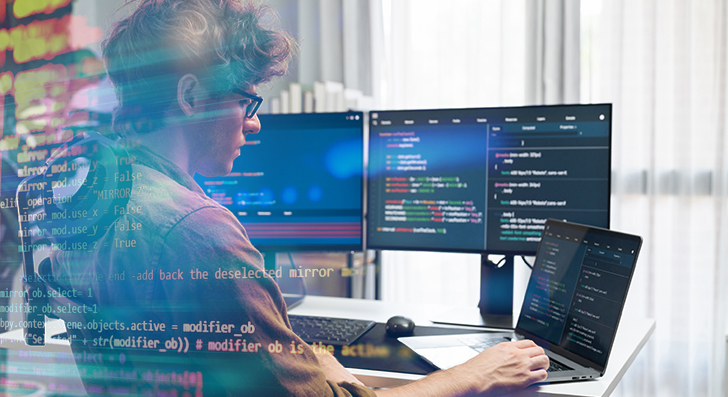
Scalability usually means your software can tackle advancement—far more consumers, more details, plus much more website traffic—with no breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic manual that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it should be part of the prepare from the start. A lot of purposes fall short every time they expand fast due to the fact the first design and style can’t deal with the additional load. As a developer, you must think early about how your process will behave under pressure.
Start off by building your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Instead, use modular layout or microservices. These styles crack your app into lesser, independent elements. Just about every module or service can scale on its own with no influencing the whole method.
Also, think of your database from day a single. Will it will need to take care of a million customers or perhaps 100? Select the suitable sort—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
One more significant issue is to prevent hardcoding assumptions. Don’t compose code that only operates beneath recent ailments. Contemplate what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that assist scaling, like concept queues or function-driven methods. These assist your app manage a lot more requests without having obtaining overloaded.
Whenever you Develop with scalability in your mind, you are not just planning for achievement—you're lessening upcoming complications. A properly-planned method is easier to take care of, adapt, and improve. It’s greater to get ready early than to rebuild afterwards.
Use the appropriate Databases
Selecting the correct databases is often a critical Section of creating scalable applications. Not all databases are developed the identical, and using the Incorrect you can sluggish you down and even trigger failures as your application grows.
Start off by knowing your information. Is it remarkably structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and regularity. They also guidance scaling strategies like read replicas, indexing, and partitioning to manage much more targeted visitors and info.
In the event your info is a lot more versatile—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and may scale horizontally extra quickly.
Also, consider your read through and generate patterns. Do you think you're doing a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a hefty publish load? Take a look at databases that may take care of superior write throughput, and even celebration-centered data storage techniques like Apache Kafka (for non permanent information streams).
It’s also wise to Consider in advance. You might not have to have Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t require to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts based upon your obtain styles. And always monitor database functionality while you increase.
In a nutshell, the best database is dependent upon your app’s construction, speed requirements, and how you anticipate it to expand. Get time to pick wisely—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every small delay provides up. Inadequately prepared code or unoptimized queries can slow down performance and overload your system. That’s why it’s imperative that you Make successful logic from the start.
Start by crafting clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated solution if a straightforward one particular functions. Keep the features limited, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also long to operate or utilizes far too much memory.
Future, examine your databases queries. These usually gradual factors down more than the code by itself. Make sure Every single query only asks for the information you truly want. Stay away from Find *, which fetches every little thing, and in its place pick precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
For those who observe the same info remaining requested repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with one hundred data could crash every time they have to handle 1 million.
In brief, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when necessary. These methods enable your software stay smooth and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to take care of more users and much more visitors. If every thing goes via 1 server, it's going to speedily turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming website traffic across several servers. As opposed to 1 server undertaking each of the function, the load balancer routes users to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to setup.
Caching is about storing data briefly so it may be reused quickly. When people request the same facts once again—like a product website page or even a profile—you don’t need to fetch it through the database each time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static information close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does transform.
In brief, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app cope with more consumers, keep fast, and Recuperate from troubles. If you propose to develop, you may need both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors improves, you can add much more resources with just a few clicks or immediately utilizing automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your application in lieu of running infrastructure.
Containers are A different essential Device. A container packages your application and all the things it ought to operate—code, libraries, options—into a single unit. This can make it effortless to move your application involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Once your application utilizes multiple containers, instruments like Kubernetes allow you to take care of them. Kubernetes handles deployment, scaling, and recovery. If a person aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container instruments signifies you are able to scale speedy, deploy simply, and recover speedily when problems come about. If you would like your application to mature without having restrictions, commence working with these resources early. They help save time, reduce possibility, and help you remain centered on constructing, not correcting.
Keep track of Anything
If you don’t keep an eye on your software, you won’t know when items go Erroneous. Checking helps you see how your app is undertaking, location problems early, and make greater selections as your application grows. It’s a key Portion of constructing scalable units.
Commence by monitoring primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just keep track of your servers—check your app way too. Control just how long it will require for people to load internet pages, how frequently glitches transpire, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a assistance goes down, it is best to get notified promptly. This will help you correct concerns quickly, frequently before users check here even see.
Checking is likewise valuable if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and facts enhance. With out checking, you’ll overlook indications of difficulties till it’s much too late. But with the best resources in place, you keep in control.
Briefly, monitoring can help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Assume big, and Create good.